Clean Coding
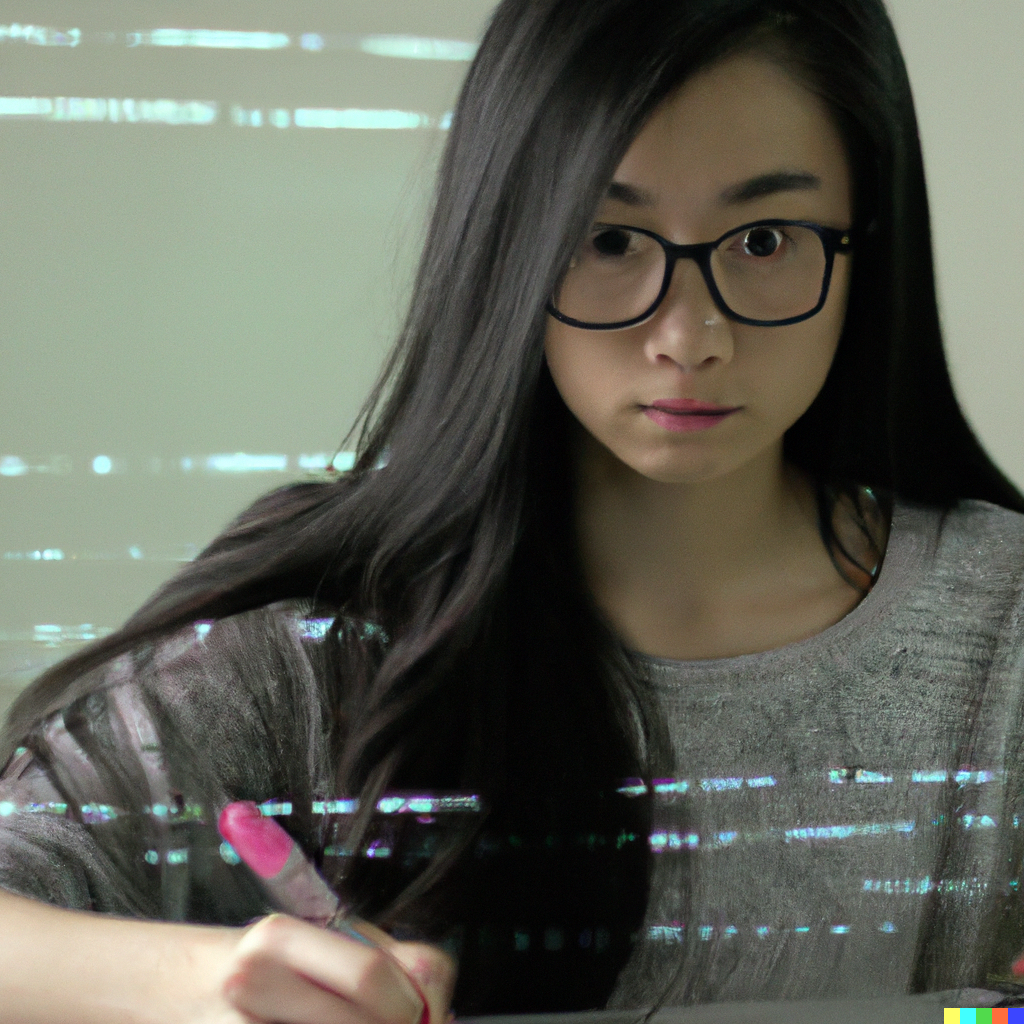
I was going to think and write about what clean coding ment to me but was struggling to do so, there’s no reason, I just couldn’t start writing. So I asked ChatGPT to do it for me. Here’s what ChatGPT wrote about clean coding:
Clean coding is a software development practice that emphasizes readability, maintainability, and simplicity. It is a way of writing code that makes it easy to understand and modify, even by someone who did not write it. Clean coding has many benefits, including improved software quality, faster development cycles, and increased developer productivity.
One of the main principles of clean coding is to write code that is easy to read and understand. This means using meaningful variable names, writing clear comments, and following a consistent coding style. When code is easy to read, it is also easier to debug and modify. This can save developers time and reduce the risk of introducing bugs into the code.
Another principle of clean coding is to write code that is maintainable. This means breaking code into small, reusable modules that can be easily modified and tested. Clean code also minimizes dependencies, making it easier to upgrade and maintain the code over time. When code is maintainable, it is also more scalable, meaning it can handle larger workloads and more complex functionality.
Clean coding can also affect performance. Writing clean code can improve the performance of an application by reducing the amount of unnecessary or redundant code. When code is clean, it is also easier to optimize, meaning developers can identify and fix performance issues more quickly. Clean code is also less likely to contain bugs, which can slow down an application or cause it to crash.
In addition to improving performance, clean coding can also have a positive impact on developer productivity. When code is clean, developers spend less time debugging and more time developing new features. Clean code is also easier to test, meaning developers can write automated tests to catch bugs before they make it into production.
In conclusion, clean coding is an essential practice for software development. It promotes readability, maintainability, and simplicity, which can lead to improved software quality, faster development cycles, and increased developer productivity. It also has a positive impact on performance by reducing unnecessary or redundant code and making it easier to optimize. Whether you are a seasoned developer or just starting out, writing clean code should be a top priority.
Well, why would anyone write about anything anymore unless it’s something very specific and unknown. From ChatGPT I see that clean coding is a well established concept. I feel so because I agree with most of the things it says.
I want to comment on what it says about improving performance. I feel this might not be the case in some cases and is really debatable. For example, in an interpreted language, when you divide your code to many classes and functions/methods to make it more readable you also make it run slower because now it has to follow classes and methods around as opposed to just interpreting a single huge function in a single file. So writing dirty code with the exact same flow will actually run faster. Here’s a very simple example demonstrating this fact:
# Apologies for the dirty code... import time def sum(x, y): return x + y LOOP_COUNT = 1000000 start = time.time() for i in range(LOOP_COUNT): my_sum = sum(1, 2) end = time.time() time_call_function = end - start print(f"Call function sum: {time_call_function}") start = time.time() for i in range(LOOP_COUNT): my_sum = 1 + 2 end = time.time() time_direct_sum = end - start print(f"Directly sum: {time_direct_sum}") print(f"{time_call_function/time_direct_sum}")
The output of this code is:
Call function sum: 0.0413360595703125
Directly sum: 0.014580011367797852
2.835118473337367
As you can see, calling the function to do the same thing a million times is 2.8 times slower than summing the values directly.
The example above doesn’t always apply to a compiled C program. At compilation, the compiler decides if there’s need to inline the function call. That means if the compiler decides it’s necessary it just replaces the function call with the body of the function. If our python code was compiled in this way, it would automatically convert the function call to the second example and the code would run 2.8 faster magically.
The point is that practicing clean coding might actually reduce performance in most cases. At that point, if you are in such a position that your code is the bottleneck of your system, you might want to think to do some things that go against clean coding.
So now let’s ask ChatGPT how can we determine if we have clean code or not?
Determining if a software code is clean code can be subjective, but there are several characteristics that are commonly agreed upon by software engineers as being indicative of clean code. Here are some guidelines that you can use to evaluate the cleanliness of a software code:
Readability: Clean code is easy to read, understand and follow. It should have clear and meaningful names for variables, functions and classes.
Simplicity: Clean code is simple and easy to understand. It follows the “Keep It Simple, Stupid” (KISS) principle, meaning that it is not overly complex or convoluted.
Modularity: Clean code is modular, meaning it is divided into small, self-contained modules that can be easily understood and tested.
Consistency: Clean code is consistent in style and structure. It follows a set of coding standards and conventions that make it easier to read and understand.
Testability: Clean code is designed to be easily testable, with clear and concise unit tests that cover all possible scenarios.
Minimalism: Clean code is minimalistic, meaning it avoids unnecessary code and follows the “Don’t Repeat Yourself” (DRY) principle.
Maintainability: Clean code is maintainable, meaning it can be easily modified and updated without introducing bugs or breaking existing functionality.
Documentation: Clean code is well-documented, with clear and concise comments and documentation that explain the purpose and functionality of each module and function.
By evaluating a software code based on these characteristics, you can get a good idea of how clean and maintainable it is. However, keep in mind that clean code is not an absolute concept and can vary depending on the specific requirements and context of the software project.
Again, I agree with almost all it says. I might not agree 100% with the documentation part where it tells to comment the code, module and function. For this part I think that the code should be self explanatory in most cases. When you have good readability you automatically have good documentation within the code. It will be easier to read and understand and this means it has built-in documentation. Of course this won’t apply to some advanced areas such as gaming, graphics, scientific software where you would expect to see a lot of advanced/complex algorithms.
In conclusion, we have some more time before AI completely takes over and we have no jobs anymore. It’s OK if the AI chooses to focus on unbelievably perfect performance with zero readability for humans. Until then, I strongly believe that focusing on clean code in a software project will pay off in a big way. Since we humans still design, plan, implement and maintain software it’s better to write code for humans to read instead of writing code for computers to run.
Leave a Reply